What Are the Best Practices for Error Handling in Php
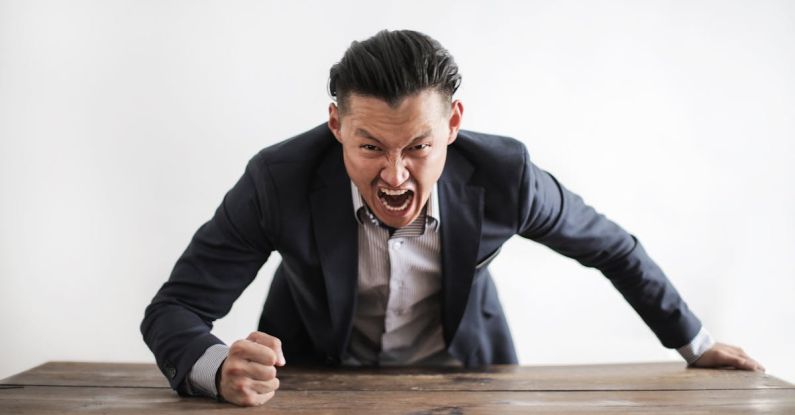
Error handling is a crucial aspect of PHP development that often gets overlooked. Properly managing errors can help you catch issues before they become bigger problems and provide a better user experience. In this article, we will explore the best practices for error handling in PHP that every developer should know.
Understanding the Basics of Error Handling in PHP
Before diving into the best practices for error handling, it’s essential to understand the basics of how errors are handled in PHP. When an error occurs in a PHP script, it triggers an error message that is displayed to the user. This can include syntax errors, runtime errors, or logical errors.
PHP provides several built-in functions for handling errors, such as `trigger_error()` and `error_reporting()`. These functions allow you to customize how errors are displayed and logged, making it easier to track down and fix issues in your code.
Best Practices for Error Reporting
One of the first steps in effective error handling is setting up proper error reporting in your PHP environment. By default, PHP may not display all errors, which can make debugging more challenging. To ensure that you catch all errors, it’s recommended to set the error reporting level to `E_ALL` in your PHP configuration file.
“`php
error_reporting(E_ALL);
“`
This setting will display all errors, warnings, and notices, giving you a comprehensive view of any issues in your code. Additionally, you can configure PHP to log errors to a file using the `log_errors` and `error_log` directives in your php.ini file.
Custom Error Handling Functions
While PHP provides built-in error handling functions, you can also create custom error handling functions to handle errors in a more structured way. By defining custom error handlers using `set_error_handler()`, you can centralize error handling logic and provide more detailed error messages to users.
“`php
function customErrorHandler($errno, $errstr, $errfile, $errline) {
// Custom error handling logic
}
set_error_handler(“customErrorHandler”);
“`
Custom error handlers give you more control over how errors are handled and allow you to log errors, send notifications, or display user-friendly messages based on the type of error.
Using Exceptions for Error Handling
Another best practice for error handling in PHP is to use exceptions for handling errors in your code. Exceptions provide a structured way to handle errors and allow you to gracefully recover from exceptional situations.
When an error occurs, you can throw an exception using the `throw` keyword and catch it using a `try…catch` block. This allows you to handle errors without disrupting the flow of your application.
“`php
try {
// Code that may throw an exception
} catch (Exception $e) {
// Handle the exception
}
“`
By using exceptions, you can differentiate between different types of errors and handle them appropriately, improving the robustness of your code.
Logging and Monitoring Errors
In addition to displaying errors to users, it’s essential to log and monitor errors in your PHP applications. Logging errors to a file or a database can help you track down recurring issues and identify patterns in your code that may be causing errors.
Using a logging library like Monolog or logging errors to a centralized system like Elasticsearch or Splunk can provide insights into the health of your application and help you proactively address issues before they impact users.
Testing Error Handling
Testing is an essential part of error handling in PHP. Writing unit tests for your error handling functions and scenarios can help you ensure that errors are handled correctly and that your application behaves as expected in exceptional situations.
By creating test cases that simulate different error conditions, you can verify that your error handling logic works as intended and provides the appropriate feedback to users.
Conclusion: Embracing Effective Error Handling in PHP
In conclusion, error handling is a critical aspect of PHP development that can significantly impact the reliability and usability of your applications. By following best practices such as setting up proper error reporting, using custom error handling functions, leveraging exceptions, and logging errors, you can improve the robustness of your code and provide a better user experience.
Implementing these best practices in your PHP projects will help you catch and address errors more effectively, leading to more stable and reliable applications. Remember that error handling is not just about fixing bugs but also about ensuring that your code is resilient and can gracefully handle unexpected situations. By embracing effective error handling techniques, you can write better PHP code and deliver higher-quality applications to your users.