How to Write Clean and Maintainable Php Code
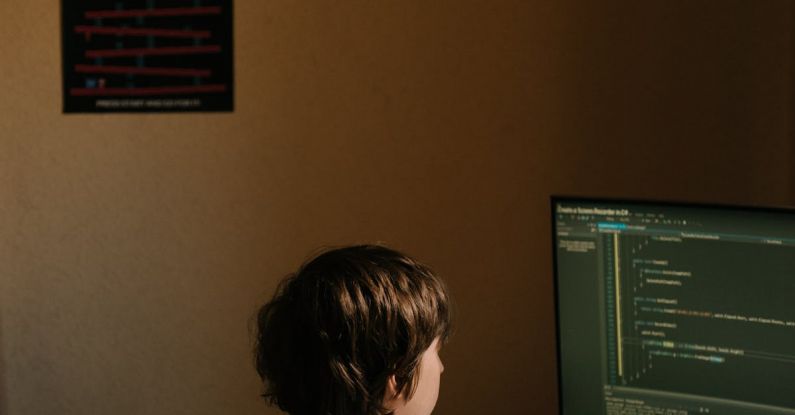
Writing clean and maintainable PHP code is essential for the efficiency and readability of your projects. In the fast-paced world of web development, having a well-organized codebase can save you time and effort in the long run. By following some best practices and guidelines, you can ensure that your PHP code is clean, easy to understand, and maintainable for future updates and modifications.
### Use Meaningful Variable Names
One of the fundamental principles of writing clean PHP code is using meaningful variable names. Instead of using vague names like $a or $temp, opt for descriptive names that convey the purpose of the variable. For example, instead of $user, you could use $loggedInUser. This not only makes your code more readable but also helps you and other developers understand its functionality at a glance.
### Follow Coding Standards
Adhering to coding standards is crucial for maintaining consistency across your codebase. Whether you follow the PSR standards or your own set of guidelines, make sure to stick to them throughout your project. Consistent indentation, naming conventions, and formatting help in making your code more readable and maintainable. Tools like PHP_CodeSniffer can be used to automatically check your code against coding standards.
### Break Down Your Code into Small Functions
Writing long, monolithic blocks of code can make your application harder to maintain and debug. Instead, break down your code into small, focused functions that perform a single task. This not only makes your code more modular but also allows for easier testing and reuse of code. Aim for functions that are concise and do one thing well.
### Comment Your Code Wisely
While it’s essential to write self-explanatory code, adding comments can provide additional context and explanations where needed. Avoid over-commenting your code, but include comments for complex algorithms, tricky logic, or sections that might be confusing to others. Comments should be clear, concise, and add value to the understanding of the code.
### Avoid Global Variables
Global variables can lead to unexpected side effects and make your code harder to reason about. Instead, encapsulate your code in classes and use object-oriented programming principles to manage state and behavior. By limiting the scope of your variables and functions, you can prevent conflicts and make your code more maintainable.
### Validate and Sanitize Input Data
Security is a critical aspect of writing PHP code, and validating and sanitizing input data is a must to prevent vulnerabilities like SQL injection and cross-site scripting attacks. Always validate user input before processing it and sanitize data before using it in queries or output. Use PHP’s built-in functions like filter_var() and htmlspecialchars() to ensure data integrity and security.
### Keep Your Code DRY
The DRY (Don’t Repeat Yourself) principle is a fundamental concept in software development that advocates for code reusability and avoiding duplication. If you find yourself repeating the same code in multiple places, consider refactoring it into a reusable function or class. By keeping your code DRY, you can reduce redundancy, improve maintainability, and make future changes easier.
### Test Your Code
Testing is an integral part of writing clean and maintainable PHP code. Whether you choose unit testing, integration testing, or end-to-end testing, having a robust testing strategy can help you catch bugs early and ensure the reliability of your code. Tools like PHPUnit and Codeception can assist you in writing and running tests to validate your code’s functionality.
### Embrace Continuous Integration
Continuous Integration (CI) is a development practice that involves regularly integrating code changes into a shared repository and automating build and testing processes. By integrating CI into your workflow, you can catch integration issues early, maintain code quality, and ensure that your codebase is always in a deployable state. Popular CI tools like Jenkins, Travis CI, and GitLab CI can help streamline your development pipeline.
### Conclusion
Writing clean and maintainable PHP code is not just about following best practices but also about fostering a mindset of quality and efficiency in your development process. By using meaningful variable names, following coding standards, breaking down code into small functions, and embracing testing and CI practices, you can create a codebase that is easy to understand, modify, and maintain. Remember, clean code is not just a one-time effort but an ongoing commitment to excellence in your development projects.