How to Use Mongodb with Php
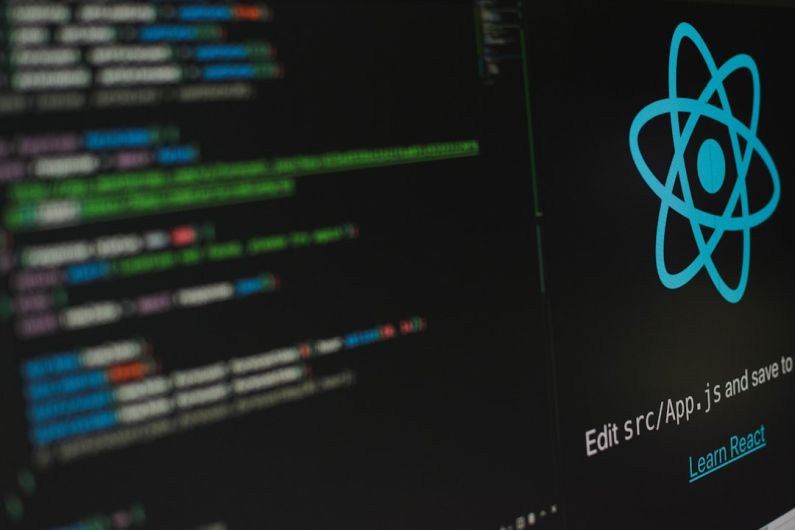
If you’re looking to harness the power of MongoDB in your PHP projects, you’re in the right place. In this article, we’ll dive into the seamless integration of MongoDB with PHP, exploring the steps to set up and use this dynamic duo for efficient data management. Let’s get started!
### Setting Up MongoDB with PHP
Before we delve into the practical implementation, it’s essential to ensure that MongoDB is installed on your system. You can download and install MongoDB from the official website based on your operating system. Once MongoDB is up and running, you’ll need to install the MongoDB PHP driver to establish a connection between PHP and MongoDB.
### Installing the MongoDB PHP Driver
To install the MongoDB PHP driver, you can utilize the Composer package manager. Open your terminal and run the following command:
“`bash
composer require mongodb/mongodb
“`
This command will fetch and install the MongoDB PHP driver for your project. Once the installation is complete, you’re ready to start coding with MongoDB in PHP.
### Connecting to MongoDB
To establish a connection to your MongoDB database within a PHP script, you need to initialize a MongoDB client object. Here’s a simple example to connect to a local MongoDB server:
“`php
require ‘vendor/autoload.php’;
use MongoDB\Client;
$client = new Client(‘mongodb://localhost:27017’);
echo “Connected successfully to MongoDB”;
“`
In this code snippet, we first include the Composer autoload file to load the MongoDB PHP driver. Next, we create a new instance of the MongoDB client by passing the MongoDB connection string as a parameter. If the connection is successful, the message “Connected successfully to MongoDB” will be displayed.
### Performing CRUD Operations
With the connection established, you can now perform Create, Read, Update, and Delete (CRUD) operations on your MongoDB database using PHP. Let’s take a look at some basic CRUD operations:
#### Inserting Data
To insert a document into a MongoDB collection, you can use the `insertOne()` method. Here’s an example:
“`php
$collection = $client->mydatabase->mycollection;
$result = $collection->insertOne([‘name’ => ‘John Doe’, ‘age’ => 30]);
echo “Document inserted with ID: ” . $result->getInsertedId();
“`
In this snippet, we first select the target collection within the database. Then, we insert a new document with the specified fields. The `getInsertedId()` method returns the unique identifier of the inserted document.
#### Querying Data
Retrieving documents from a MongoDB collection involves using the `find()` method. Here’s a simple example:
“`php
$collection = $client->mydatabase->mycollection;
$cursor = $collection->find();
foreach ($cursor as $document) {
echo $document[‘name’] . ‘ – ‘ . $document[‘age’] . ‘
‘;
}
“`
In this code snippet, we fetch all documents from the collection and iterate over the result set to display the document fields.
#### Updating Data
To update a document in a MongoDB collection, you can use the `updateOne()` method. Here’s an example:
“`php
$collection = $client->mydatabase->mycollection;
$result = $collection->updateOne([‘name’ => ‘John Doe’], [‘$set’ => [‘age’ => 35]]);
echo “Document updated successfully”;
“`
This code snippet updates the ‘age’ field of the document where the ‘name’ is ‘John Doe’.
#### Deleting Data
Deleting a document from a MongoDB collection can be done using the `deleteOne()` method. Here’s an example:
“`php
$collection = $client->mydatabase->mycollection;
$result = $collection->deleteOne([‘name’ => ‘John Doe’]);
echo “Document deleted successfully”;
“`
In this snippet, we remove the document where the ‘name’ is ‘John Doe’ from the collection.
### Enhancing Performance with Indexing
Indexing plays a crucial role in optimizing database queries for faster retrieval of data. By creating indexes on fields frequently used in queries, you can significantly improve the performance of your MongoDB database.
To create an index on a specific field, you can use the `createIndex()` method. Here’s an example:
“`php
$collection = $client->mydatabase->mycollection;
$collection->createIndex([‘name’ => 1]);
echo “Index created successfully on the ‘name’ field”;
“`
By indexing the ‘name’ field in this example, you can accelerate queries that involve filtering or sorting based on the ‘name’ attribute.
### Securing Your MongoDB Connection
When connecting to a MongoDB server from PHP, it’s essential to ensure the security of your database connection. You can enhance the security of your MongoDB deployment by enabling authentication and configuring user roles and permissions.
To authenticate your connection to MongoDB, you can specify the username and password in the connection string:
“`php
$client = new Client(‘mongodb://username:password@localhost:27017’);
“`
By providing valid credentials in the connection string, you can authenticate your PHP script with the MongoDB server.
### Conclusion: Harnessing the Power of MongoDB with PHP
In conclusion, integrating MongoDB with PHP opens up a world of possibilities for efficient data storage and retrieval in your web applications. By following the steps outlined in this article, you can seamlessly connect to MongoDB, perform CRUD operations, optimize performance with indexing, and secure your database connection.
Embrace the flexibility and scalability offered by MongoDB and PHP to build robust and dynamic applications that meet the demands of modern web development. Start exploring the endless possibilities of this powerful combination and elevate your projects to the next level. Happy coding!