How to Use Guzzle for Http Requests
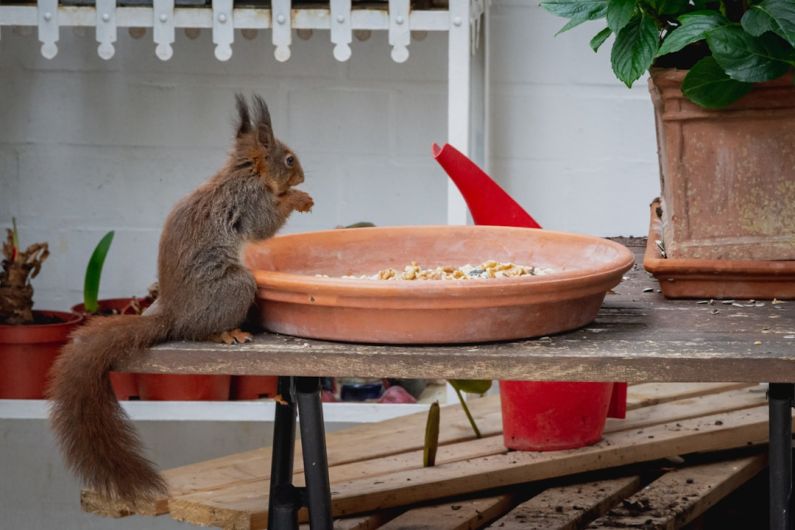
Making HTTP requests is a common task in web development, and the Guzzle library provides a powerful and flexible way to handle this task in PHP. In this article, we will explore how to use Guzzle for HTTP requests efficiently and effectively.
### What is Guzzle?
Guzzle is a PHP HTTP client library that makes it easy to send HTTP requests and integrate with web services. It simplifies the process of making requests, handling responses, and interacting with APIs. Guzzle is widely used in PHP projects for its robust feature set and ease of use.
### Installation
To start using Guzzle in your PHP project, you first need to install it using Composer. If you haven’t already installed Composer, you can do so by following the instructions on the official Composer website. Once Composer is set up, you can install Guzzle by running the following command in your project directory:
“`
composer require guzzlehttp/guzzle
“`
This command will download and install Guzzle and all its dependencies in your project.
### Making GET Requests
One of the most common types of HTTP requests is the GET request, which is used to retrieve data from a server. With Guzzle, making a GET request is straightforward. Here’s an example:
“`php
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request(‘GET’, ‘https://api.example.com/data’);
echo $response->getBody();
“`
In this example, we create a new Guzzle Client instance and use it to make a GET request to `https://api.example.com/data`. The response object contains the data returned by the server, which we can then output or process as needed.
### Making POST Requests
In addition to GET requests, Guzzle also supports other HTTP methods like POST for sending data to a server. Here’s how you can make a POST request using Guzzle:
“`php
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request(‘POST’, ‘https://api.example.com/data’, [
‘json’ => [‘key’ => ‘value’]
]);
echo $response->getBody();
“`
In this example, we make a POST request to `https://api.example.com/data` and include some JSON data in the request body. The server will process this data and return a response, which we can then handle accordingly.
### Handling Responses
When making HTTP requests with Guzzle, you will often need to handle the responses returned by the server. The response object provides various methods for accessing different parts of the response, such as the body, headers, and status code. Here’s an example of how you can handle a response in Guzzle:
“`php
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request(‘GET’, ‘https://api.example.com/data’);
echo $response->getStatusCode(); // Output the status code
echo $response->getBody(); // Output the response body
“`
In this example, we make a GET request and then use the `getStatusCode()` and `getBody()` methods of the response object to access the status code and body of the response, respectively.
### Error Handling
Error handling is an essential aspect of making HTTP requests, as things don’t always go as planned. Guzzle provides mechanisms for handling errors that occur during request processing. One common approach is to use try-catch blocks to catch exceptions thrown by Guzzle. Here’s an example:
“`php
use GuzzleHttp\Client;
use GuzzleHttp\Exception\RequestException;
$client = new Client();
try {
$response = $client->request(‘GET’, ‘https://api.example.com/data’);
echo $response->getBody();
} catch (RequestException $e) {
echo $e->getMessage();
}
“`
In this example, we attempt to make a GET request, but if an error occurs during the request, a `RequestException` will be thrown. We catch this exception and handle it accordingly, such as by outputting an error message.
### Summary
In this article, we’ve explored how to use Guzzle for making HTTP requests in PHP. Guzzle simplifies the process of sending requests, handling responses, and interacting with web services. By following the examples and guidelines provided, you can effectively use Guzzle in your PHP projects to communicate with servers and APIs. Happy coding!