How to Use Encryption in Php
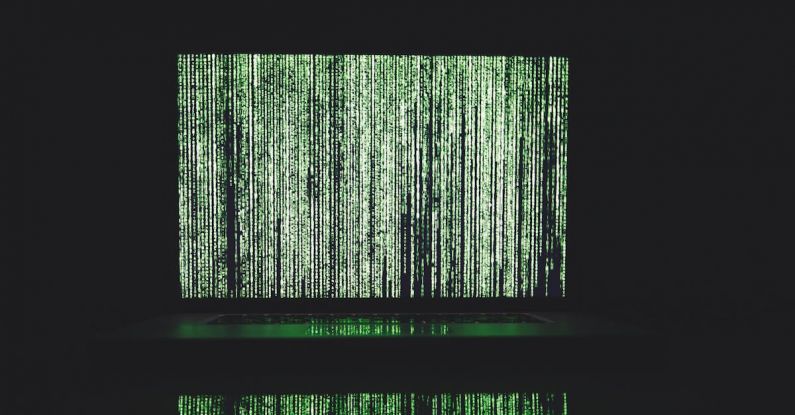
In today’s digital age, the security of sensitive information is of paramount importance. As a PHP developer, it is essential to understand how encryption can be utilized to protect data from unauthorized access. Encryption is the process of converting data into a secure format that can only be read by individuals with the proper decryption key. In this article, we will explore how to effectively use encryption in PHP to safeguard your data.
### Understanding Encryption
Before diving into how to implement encryption in PHP, it is crucial to understand the basics of encryption. Encryption relies on algorithms to scramble data into a format that is unreadable without the corresponding decryption key. There are two primary types of encryption: symmetric and asymmetric.
### Symmetric Encryption
Symmetric encryption involves using the same key for both encryption and decryption processes. This means that whoever has the key can encrypt and decrypt the data. In PHP, the `openssl_encrypt` and `openssl_decrypt` functions can be used to implement symmetric encryption. It is important to securely store and manage the encryption key to prevent unauthorized access to the encrypted data.
### Asymmetric Encryption
Asymmetric encryption, also known as public-key encryption, utilizes a pair of keys: a public key for encryption and a private key for decryption. The public key can be shared with anyone, while the private key must be kept secure. PHP supports asymmetric encryption through the `openssl_public_encrypt` and `openssl_private_decrypt` functions. Asymmetric encryption is commonly used for secure communication and digital signatures.
### Implementing Encryption in PHP
To implement encryption in PHP, follow these steps:
1. **Generate Encryption Keys**: For symmetric encryption, generate a secure encryption key using the `openssl_random_pseudo_bytes` function. For asymmetric encryption, generate a public-private key pair using the `openssl_pkey_new` function.
2. **Encrypt Data**: Use the appropriate encryption function (`openssl_encrypt` for symmetric encryption or `openssl_public_encrypt` for asymmetric encryption) to encrypt the data with the encryption key.
3. **Store Encrypted Data**: Store the encrypted data in a secure location, such as a database or file system. Ensure that access to the encrypted data is restricted to authorized users only.
4. **Decrypt Data**: When retrieving the encrypted data, use the corresponding decryption function (`openssl_decrypt` for symmetric encryption or `openssl_private_decrypt` for asymmetric encryption) with the decryption key to decrypt the data.
### Best Practices for Encryption in PHP
When using encryption in PHP, it is important to follow best practices to ensure the security of your data:
– **Use Strong Encryption Algorithms**: Choose encryption algorithms that are considered secure and robust, such as AES for symmetric encryption and RSA for asymmetric encryption.
– **Secure Key Management**: Safeguard encryption keys by storing them securely and restricting access to authorized users only. Consider using key management services for added security.
– **Protect Against Attacks**: Implement measures to protect against common attacks, such as brute force attacks and padding oracle attacks. Use secure coding practices to prevent vulnerabilities in your encryption implementation.
– **Regularly Update Encryption Methods**: Stay updated on the latest encryption techniques and best practices. Regularly review and update your encryption methods to mitigate emerging security threats.
### Safeguarding Your Data with Encryption
By understanding how encryption works and following best practices for implementing encryption in PHP, you can effectively safeguard your data from unauthorized access. Encryption plays a crucial role in protecting sensitive information and ensuring the security and confidentiality of your data. Stay vigilant, stay informed, and stay secure in the ever-evolving landscape of cybersecurity.