How to Manage Dependency Injection with Php-di
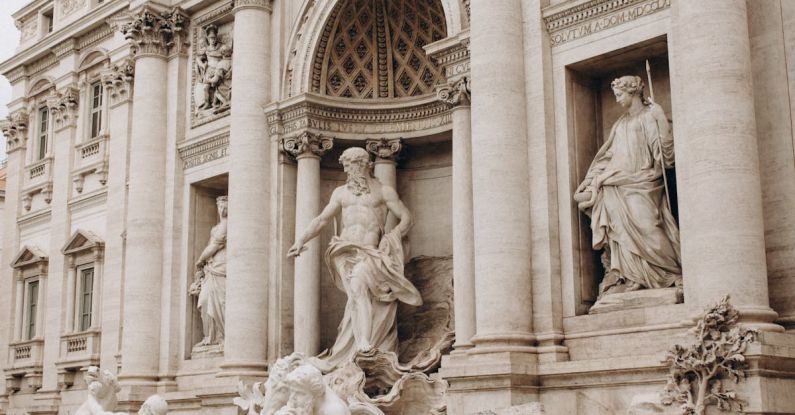
Dependency injection is a crucial aspect of modern software development, allowing developers to write more modular and maintainable code. When it comes to managing dependency injection in PHP applications, php-di (PHP-DI) is a powerful and flexible tool that simplifies the process. In this article, we will explore how to effectively use php-di to manage dependencies in your PHP projects.
Understanding Dependency Injection
Before diving into how to manage dependency injection with php-di, it’s essential to understand the concept of dependency injection itself. Dependency injection is a design pattern that involves passing objects (dependencies) into a class rather than having the class create them itself. This approach promotes loose coupling between components and makes it easier to test and reuse code.
Installing php-di
The first step in managing dependency injection with php-di is to install the library. You can easily install php-di using Composer by running the following command in your project directory:
“`bash
composer require php-di/php-di
“`
Defining Dependencies
Once php-di is installed, you need to define your dependencies. In php-di, dependencies are defined in a PHP configuration file. You can specify dependencies using class names or interfaces, along with any parameters required for object instantiation.
“`php
return [
‘MyService’ => DI\create()
->constructor(DI\get(‘Dependency1’), DI\get(‘Dependency2’))
];
“`
In this example, ‘MyService’ is defined with dependencies ‘Dependency1’ and ‘Dependency2’. When ‘MyService’ is instantiated, php-di will automatically inject the required dependencies.
Injecting Dependencies
To use dependency injection in your classes, you need to configure the php-di container and retrieve instances of your services. You can create an instance of the container and retrieve services as follows:
“`php
$container = new DI\ContainerBuilder();
$container = $container->build();
$myService = $container->get(‘MyService’);
“`
By retrieving services from the container, php-di will handle the injection of dependencies for you. This simplifies the process of managing dependencies and ensures that your classes are loosely coupled and easy to test.
Scopes and Lazy Loading
Php-di supports different scopes for dependencies, allowing you to control when objects are created and how long they exist. By default, dependencies are created in the ‘prototype’ scope, meaning a new instance is created each time the dependency is injected.
You can specify a different scope for a dependency by using the ‘scope’ method:
“`php
return [
‘MyService’ => DI\create()
->constructor(DI\get(‘Dependency1’), DI\get(‘Dependency2’))
->scope(DI\Scope::SINGLETON)
];
“`
In this example, ‘MyService’ is defined as a singleton, meaning only one instance will be created and reused each time it is injected.
Testing Dependencies
One of the key benefits of using dependency injection is that it makes testing easier. With php-di, you can easily mock dependencies in your tests by defining different configurations for the container:
“`php
$testContainer = new DI\ContainerBuilder();
$testContainer = $testContainer->addDefinitions([
‘Dependency1’ => DI\create(MockDependency::class)
]);
$myService = $testContainer->build()->get(‘MyService’);
“`
By defining mock dependencies in your test container, you can isolate the code you are testing and ensure that it behaves as expected without relying on external dependencies.
Optimizing Performance
While dependency injection is a powerful tool, it can impact performance if not used carefully. Php-di offers features like lazy loading and proxy objects to optimize performance by only creating objects when they are needed.
By using lazy loading and scoping dependencies appropriately, you can ensure that your application remains performant while still benefiting from the flexibility and maintainability of dependency injection.
Incorporating php-di into your PHP projects can significantly improve the structure and maintainability of your codebase. By following best practices for managing dependencies, you can write more modular, testable, and efficient code that is easier to maintain and scale.
Embracing Dependency Injection with php-di
As you continue to explore the world of dependency injection in PHP, php-di stands out as a valuable tool for managing dependencies in your projects. By understanding the core principles of dependency injection, defining dependencies, injecting them into your classes, and optimizing performance, you can leverage php-di to write clean, maintainable, and scalable code.