How to Integrate Slim Framework with Your Project
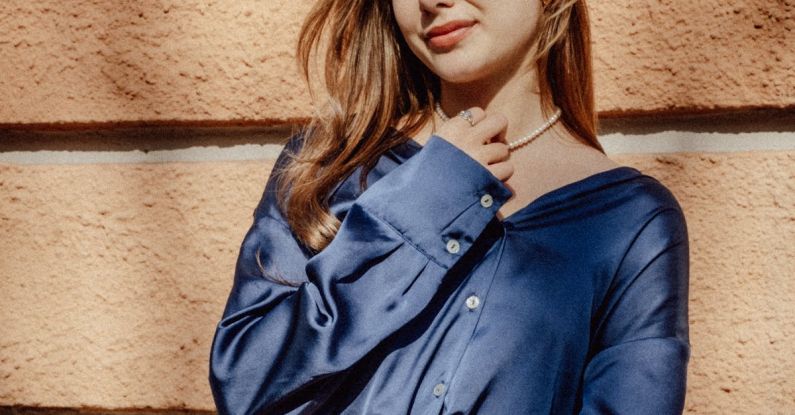
Slim Framework is a lightweight PHP micro-framework that allows developers to quickly build powerful web applications and APIs. Integrating Slim Framework with your project can streamline the development process and make your code more maintainable. In this article, we will explore how you can easily integrate Slim Framework into your project.
Getting Started with Slim Framework
Before you can integrate Slim Framework into your project, you need to install it. The easiest way to do this is using Composer, a dependency manager for PHP. Simply create a new project directory and run the following command in your terminal:
“`bash
composer require slim/slim
“`
This will download and install the latest version of Slim Framework into your project. Once Slim Framework is installed, you can start building your application.
Creating Routes
One of the key features of Slim Framework is its routing capabilities. Routes are used to define how your application responds to incoming HTTP requests. To create a new route in Slim Framework, you can use the following syntax:
“`php
use Slim\Factory\AppFactory;
require __DIR__ . ‘/vendor/autoload.php’;
$app = AppFactory::create();
$app->get(‘/hello/{name}’, function ($request, $response, $args) {
$name = $args[‘name’];
$response->getBody()->write(“Hello, $name”);
return $response;
});
$app->run();
“`
In this example, we have defined a GET route that responds with a simple greeting message. You can define routes for various HTTP methods such as GET, POST, PUT, DELETE, etc., and handle different types of requests in your application.
Middleware
Middleware is another powerful feature of Slim Framework that allows you to modify the request and response objects as they pass through the application. Middleware functions can be used for tasks such as authentication, logging, error handling, and more. You can add middleware to your Slim application using the following syntax:
“`php
$app->add(function ($request, $handler) {
$response = $handler->handle($request);
$response->getBody()->write(‘Middleware Example’);
return $response;
});
“`
In this example, we have added a simple middleware function that appends a message to the response body. You can create custom middleware functions to suit the needs of your application and add them to the application stack.
Error Handling
Handling errors gracefully is essential for any web application. Slim Framework provides a convenient way to handle errors using its error handling middleware. You can define error handling middleware in Slim Framework as follows:
“`php
$errorMiddleware = $app->addErrorMiddleware(true, true, true);
“`
This will enable the error handling middleware in Slim Framework and display detailed error messages when an exception occurs in your application. You can customize the error handling behavior to suit your requirements.
Conclusion
Integrating Slim Framework into your project can help you build robust and maintainable web applications and APIs. By following the simple steps outlined in this article, you can quickly get started with Slim Framework and take advantage of its powerful features. Start integrating Slim Framework into your project today and experience the benefits of this lightweight PHP micro-framework.