How to Implement Image Manipulation with Imagine
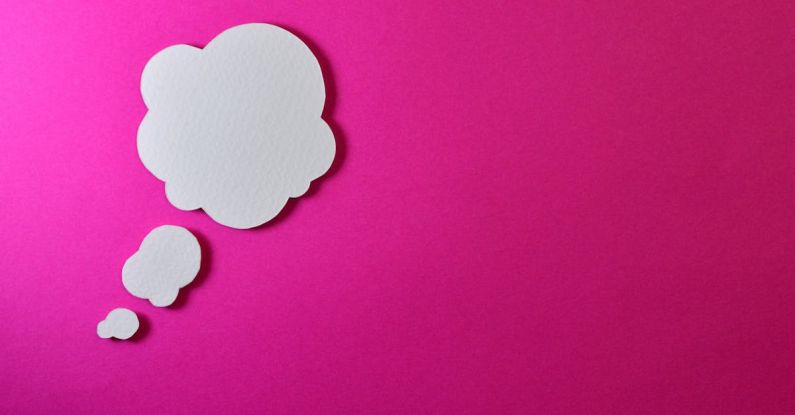
Image manipulation is a crucial aspect of modern web development and graphic design. It allows developers and designers to enhance, edit, and transform images to better suit their needs. One powerful tool for implementing image manipulation in PHP applications is Imagine. Imagine is a PHP library that provides a simple and intuitive API for working with images. In this article, we will explore how to effectively implement image manipulation with Imagine.
Getting Started with Imagine
To begin using Imagine in your PHP project, you first need to install the library using Composer. Composer is a dependency management tool for PHP that simplifies the process of installing and managing libraries and packages. Once you have Composer set up in your project, you can install Imagine by running the following command in your terminal:
“`
composer require imagine/imagine
“`
Once Imagine is installed, you can start using its powerful features to manipulate images in your PHP applications.
Loading and Manipulating Images
Imagine provides a straightforward way to load images from files and manipulate them using a variety of filters and effects. To load an image from a file, you can use the `open` method:
“`php
use Imagine\Gd\Imagine;
$imagine = new Imagine();
$image = $imagine->open(‘path/to/image.jpg’);
“`
Once you have loaded an image, you can apply various manipulations to it, such as resizing, cropping, rotating, and applying filters. Imagine uses a fluent interface that allows you to chain multiple operations together to create complex image manipulations. For example, to resize an image and apply a grayscale filter, you can do the following:
“`php
$image->resize(new Box(200, 200))
->effects()
->grayscale();
“`
Saving and Displaying Images
After you have manipulated an image to your liking, you can save it to a file or output it directly to the browser. Imagine provides methods for saving images in various formats, such as JPEG, PNG, and GIF. To save an image to a file, you can use the `save` method:
“`php
$image->save(‘path/to/output.jpg’);
“`
If you want to display the manipulated image directly in a web page, you can output it to the browser using the `show` method:
“`php
header(‘Content-Type: image/jpeg’);
echo $image->show(‘jpg’);
“`
By using Imagine’s simple API, you can easily load, manipulate, save, and display images in your PHP applications with just a few lines of code.
Applying Filters and Effects
Imagine provides a wide range of filters and effects that you can apply to images to achieve various visual effects. These include blur, sharpen, negate, grayscale, and many more. By combining multiple filters and effects, you can create unique and visually appealing images for your web projects. For example, you can apply a blur effect followed by a grayscale filter to create a stylish and artistic look:
“`php
$image->effects()
->blur(10)
->grayscale();
“`
Experimenting with different filters and effects is a great way to unleash your creativity and enhance the visual impact of your images.
Handling Image Formats and Quality
When working with images in PHP applications, it is essential to consider the image format and quality to ensure optimal performance and visual fidelity. Imagine allows you to specify the output format and quality when saving images, giving you full control over the final result. You can set the output format and quality like this:
“`php
$image->save(‘path/to/output.jpg’, [‘jpeg_quality’ => 90]);
“`
By adjusting the image format and quality settings, you can optimize the size and visual appearance of your images for different use cases.
Incorporating Image Manipulation into Your Projects
Implementing image manipulation with Imagine can greatly enhance the visual appeal and functionality of your PHP applications. Whether you are building a website, a web application, or a graphic design tool, Imagine provides a powerful and flexible solution for working with images. By following the tips and techniques outlined in this article, you can leverage Imagine’s features to create stunning images that captivate your audience and elevate your projects to new heights.