How to Handle Exceptions in Php
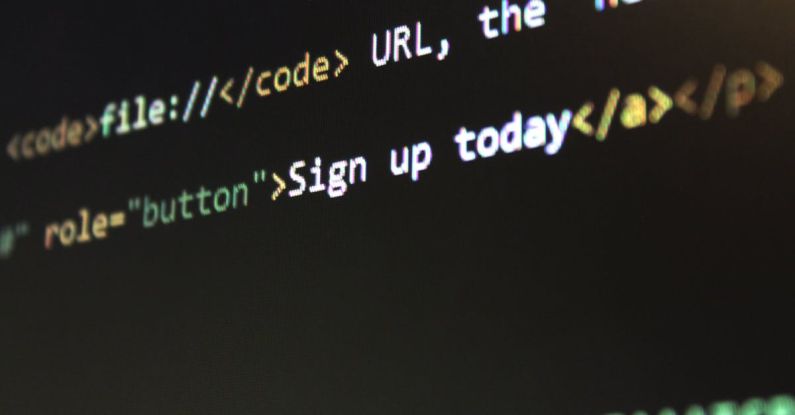
Exception handling is a crucial aspect of any PHP application development process. When writing code, it’s essential to anticipate and handle exceptions that may occur during the execution of the program. By effectively managing exceptions, developers can ensure that their applications remain stable and provide a better user experience. In this article, we will explore how to handle exceptions in PHP efficiently.
### What are Exceptions in PHP?
Before delving into how to handle exceptions, let’s first understand what exceptions are in PHP. An exception is an event that disrupts the normal flow of a program’s execution. When an exception occurs, the PHP interpreter looks for an appropriate exception handler to deal with it. If no handler is found, the program terminates, and an error message is displayed to the user.
### Using Try…Catch Blocks
One of the primary ways to handle exceptions in PHP is by using try…catch blocks. The try block contains the code that might throw an exception, while the catch block is used to handle the exception if it occurs. By encapsulating the potentially problematic code within a try block, developers can gracefully manage any exceptions that arise.
### Creating Custom Exception Classes
In addition to using built-in PHP exceptions, developers can also create custom exception classes to handle specific types of errors. By extending the base `Exception` class, developers can define their own exception types with custom error messages and properties. This allows for more granular control over how different types of exceptions are handled within the application.
### Rethrowing Exceptions
Sometimes it may be necessary to catch an exception, perform some operations, and then rethrow the same exception or a different one. This can be achieved by using the `throw` statement within a catch block. Rethrowing exceptions can be useful in scenarios where additional processing or logging needs to be done before passing the exception up the call stack.
### Using Finally Blocks
The finally block in a try…catch structure is used to define code that should be executed regardless of whether an exception occurs. This block is useful for performing cleanup operations, such as releasing resources or closing database connections, that need to be executed regardless of the outcome of the try block.
### Handling Multiple Exceptions
In some cases, a single block of code may throw multiple exceptions of different types. To handle this situation, developers can use multiple catch blocks, each corresponding to a different exception type. By specifying multiple catch blocks, developers can implement different handling logic for each type of exception that may be thrown.
### Best Practices for Exception Handling
When it comes to handling exceptions in PHP, there are several best practices that developers should keep in mind. One important practice is to provide informative error messages that help users understand what went wrong. Additionally, it’s essential to log exceptions to facilitate troubleshooting and debugging.
### Conclusion: Ensuring Robust PHP Applications
In conclusion, effective exception handling is crucial for ensuring the robustness and reliability of PHP applications. By using try…catch blocks, creating custom exception classes, and following best practices, developers can gracefully manage exceptions and provide a better user experience. Exception handling is an essential skill for any PHP developer, and mastering it can lead to more stable and maintainable codebases.