How to Handle Database Transactions in Php
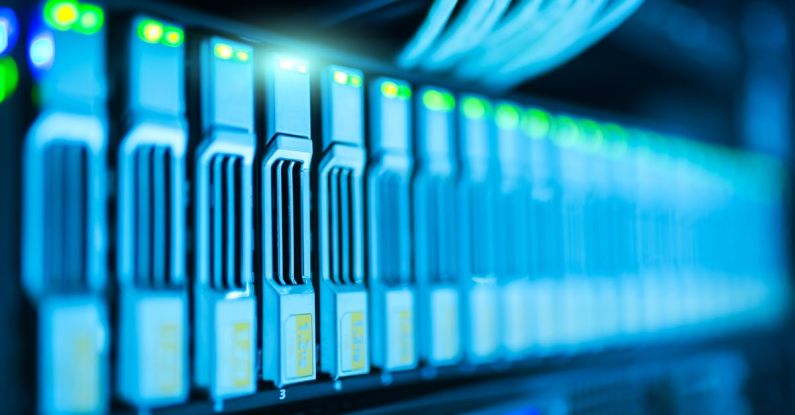
Databases play a crucial role in web development, storing and retrieving data efficiently. When working with databases in PHP, handling transactions is essential to ensure data consistency and integrity. In this article, we will delve into how to effectively manage database transactions in PHP to maintain data accuracy and reliability.
Understanding Database Transactions in PHP
Transactions in a database context refer to a sequence of operations that are treated as a single unit of work. These operations are either completed entirely or rolled back entirely if an error occurs, ensuring that the database remains in a consistent state. In PHP, transactions are typically used when executing multiple SQL queries that need to be executed together to maintain data integrity.
Starting a Transaction
To begin a transaction in PHP, you can use the following code snippet:
“`
$pdo->beginTransaction();
“`
This command initiates a transaction on the specified PDO connection. All subsequent SQL queries executed on this connection will be part of the same transaction until it is either committed or rolled back.
Committing a Transaction
Once you have executed all the necessary SQL queries within a transaction and are satisfied with the results, you can commit the transaction to make the changes permanent in the database. To commit a transaction in PHP, use the following code:
“`
$pdo->commit();
“`
This command finalizes the transaction, applying all the changes made within it to the database. After committing a transaction, the database will reflect the updated data.
Rolling Back a Transaction
In case an error occurs during the execution of SQL queries within a transaction, it is crucial to roll back the transaction to maintain data consistency. Rolling back a transaction in PHP can be done with the following code:
“`
$pdo->rollBack();
“`
By calling this command, all changes made within the transaction will be discarded, reverting the database to its state before the transaction began. This ensures that no partial or incorrect data is left in the database due to a failed transaction.
Handling Exceptions in Transactions
When working with database transactions in PHP, it is essential to handle exceptions properly to prevent unexpected behavior and ensure data integrity. By using try-catch blocks, you can capture any exceptions that may occur during the execution of SQL queries within a transaction and take appropriate actions, such as rolling back the transaction.
“`
try {
// Start transaction
$pdo->beginTransaction();
// Execute SQL queries
// Commit transaction
$pdo->commit();
} catch (PDOException $e) {
// Roll back transaction on exception
$pdo->rollBack();
// Handle the exception
echo “Error: ” . $e->getMessage();
}
“`
By wrapping your transaction code in a try-catch block, you can ensure that the transaction is rolled back if an exception occurs, preventing data inconsistencies in the database.
Optimizing Database Transactions
While transactions are essential for maintaining data integrity, it is also important to optimize them for performance. Avoid starting transactions unnecessarily or keeping them open for an extended period, as this can impact database performance.
Additionally, consider breaking down large transactions into smaller, more manageable units to reduce the risk of conflicts and improve concurrency. By optimizing your database transactions, you can ensure both data consistency and efficient database operations.
In conclusion,
Effectively handling database transactions in PHP is crucial for maintaining data consistency and integrity. By understanding how transactions work, starting, committing, rolling back transactions, handling exceptions, and optimizing transaction performance, you can ensure that your database operations are reliable and efficient. Implementing best practices for managing transactions will help you build robust and scalable web applications that rely on databases to store and retrieve data accurately.