How to Debug Database Queries in Php
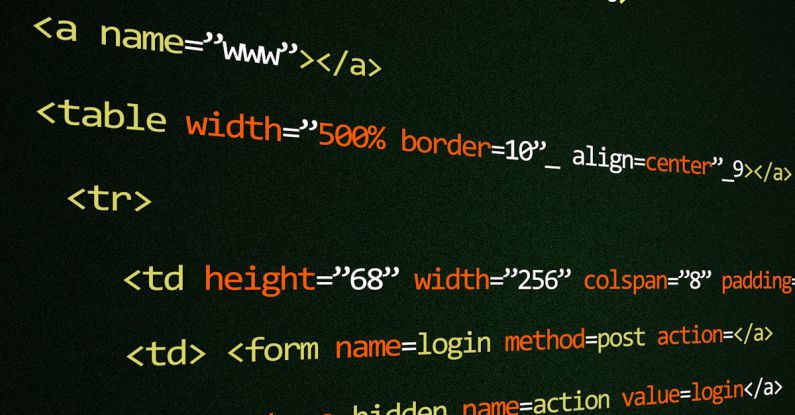
Debugging database queries in PHP is a crucial skill for any developer working with databases. Whether you’re troubleshooting slow performance, incorrect results, or just trying to understand what’s happening behind the scenes, knowing how to effectively debug your queries can save you countless hours of frustration. In this article, we’ll explore some essential techniques for debugging database queries in PHP that will help you identify and fix issues quickly.
Understanding the Query Execution Process
Before diving into specific debugging techniques, it’s essential to understand how database queries are executed in PHP. When you run a query, it goes through several steps, including parsing, optimization, execution, and fetching results. By understanding these steps, you can pinpoint where issues might be occurring and focus your debugging efforts more effectively.
Enable Error Reporting
One of the first steps in debugging database queries in PHP is to ensure that error reporting is enabled. By setting error reporting to display all errors, warnings, and notices, you’ll be able to catch any issues that arise during query execution. To enable error reporting, you can use the following code snippet at the beginning of your PHP script:
“`php
error_reporting(E_ALL);
ini_set(‘display_errors’, 1);
“`
By doing this, you’ll be able to see any errors that occur during query execution, such as syntax errors, connection issues, or other problems that may arise.
Check for Syntax Errors
Syntax errors in your SQL queries can cause them to fail silently or return unexpected results. To check for syntax errors, you can use the `mysqli_error()` function to get the error message returned by the database server. For example:
“`php
$result = mysqli_query($connection, $query);
if (!$result) {
die(‘Error: ‘ . mysqli_error($connection));
}
“`
By checking for syntax errors in this way, you can quickly identify and fix any issues that may be causing your queries to fail.
Use Prepared Statements
Prepared statements are a powerful tool for preventing SQL injection attacks and can also help with debugging database queries. By using prepared statements, you can separate the query from the data, making it easier to troubleshoot issues with your SQL syntax. Prepared statements also help improve query performance by allowing the database server to cache query execution plans.
To use prepared statements in PHP, you can use the `mysqli_prepare()` function to create a prepared statement and bind parameters to the query. For example:
“`php
$stmt = mysqli_prepare($connection, ‘SELECT * FROM users WHERE id = ?’);
mysqli_stmt_bind_param($stmt, ‘i’, $id);
mysqli_stmt_execute($stmt);
“`
By using prepared statements, you can ensure that your queries are secure and easier to debug.
Check Query Performance
Performance issues are common when working with databases, and debugging slow queries can be a challenging task. To identify performance bottlenecks in your database queries, you can use tools like MySQL’s `EXPLAIN` statement to analyze the query execution plan and identify areas for optimization.
For example, you can run the following query to get the execution plan for a given query:
“`sql
EXPLAIN SELECT * FROM users WHERE id = 1;
“`
By analyzing the output of the `EXPLAIN` statement, you can see how the database server is executing your query and identify any potential performance issues, such as missing indexes or inefficient query plans.
Monitor Database Connections
Another common issue when working with databases is managing database connections effectively. If your application is opening and closing database connections frequently, it can lead to performance issues and connection leaks. By monitoring your database connections, you can ensure that connections are being reused efficiently and closed properly when no longer needed.
You can use tools like MySQL’s `SHOW PROCESSLIST` statement to monitor active database connections and identify any connections that may be causing issues. For example:
“`sql
SHOW PROCESSLIST;
“`
By monitoring your database connections, you can identify and resolve issues related to connection management and improve the overall performance of your application.
Conclusion: Enhance Your Debugging Skills
Debugging database queries in PHP is an essential skill for any developer working with databases. By understanding the query execution process, enabling error reporting, checking for syntax errors, using prepared statements, analyzing query performance, and monitoring database connections, you can effectively identify and fix issues with your queries. By honing your debugging skills and practicing these techniques, you’ll be better equipped to troubleshoot database-related issues and build more efficient and reliable applications.