How to Create Reusable Code in Php
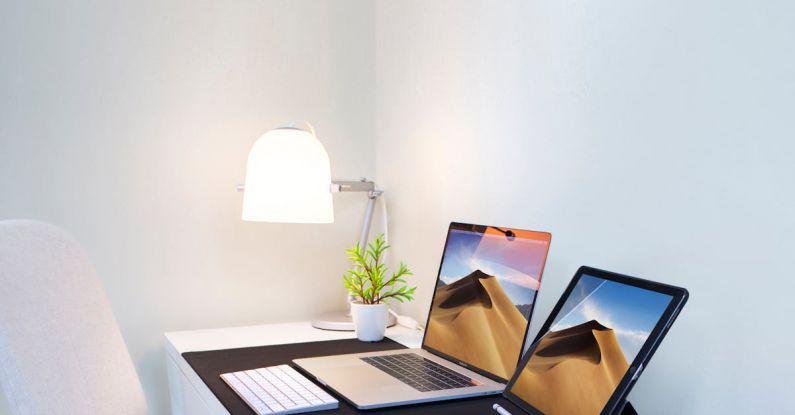
Creating reusable code in PHP is a fundamental aspect of efficient and maintainable programming. When you write code that can be easily repurposed in different parts of your application, you save time and effort in the long run. In this article, we will explore some best practices for creating reusable code in PHP that will help you write cleaner, more efficient, and easier-to-manage code.
### Use Functions Wisely
Functions are the building blocks of reusable code in PHP. By encapsulating a set of instructions within a function, you can easily call that function whenever you need to perform that specific task. When creating functions, it is essential to make them as generic and versatile as possible. Avoid hardcoding specific values within the function and instead use parameters to make the function adaptable to different scenarios.
### Embrace Object-Oriented Programming
Object-oriented programming (OOP) is a powerful paradigm that allows you to create reusable code through the use of classes and objects. By defining classes that encapsulate data and behavior, you can create instances (objects) of those classes that can be reused throughout your application. OOP promotes code reusability by enabling you to inherit properties and methods from parent classes, reducing redundancy and promoting a more organized code structure.
### Utilize Inheritance and Polymorphism
Inheritance is a key concept in OOP that enables you to create new classes based on existing ones, inheriting their properties and methods. By defining a base class with common functionality and then creating subclasses that extend or override that functionality, you can create a hierarchy of classes that promote code reuse. Polymorphism, on the other hand, allows objects of different classes to be treated as instances of a common superclass, enabling you to write code that operates on objects of varying types.
### Implement Interfaces and Traits
Interfaces and traits are additional tools in PHP that can help you create reusable code. Interfaces define a contract that classes must adhere to, specifying the methods that a class must implement. By creating interfaces for common functionality, you can ensure that different classes can share the same methods, promoting code reuse. Traits, on the other hand, allow you to define reusable sets of methods that can be included in multiple classes, providing a way to share code across different class hierarchies.
### Practice DRY (Don’t Repeat Yourself) Principle
The DRY principle is a fundamental concept in software development that emphasizes the importance of avoiding redundancy in your code. When you find yourself writing the same code in multiple places, it’s a sign that you should refactor that code into a reusable function, class, or trait. By adhering to the DRY principle, you not only make your code more maintainable but also reduce the risk of introducing bugs due to inconsistencies in duplicated code.
### Write Unit Tests
Unit testing is an essential practice in creating reusable code. By writing tests that validate the functionality of your code in isolation, you can ensure that your reusable components work as intended and can be safely integrated into different parts of your application. Unit tests also serve as documentation for how your code should behave, making it easier for other developers to understand and utilize your reusable code.
### Conclusion: Embrace Reusability for Efficient PHP Programming
Creating reusable code in PHP is a skill that every developer should strive to master. By using functions, embracing object-oriented programming, utilizing inheritance and polymorphism, implementing interfaces and traits, practicing the DRY principle, and writing unit tests, you can create code that is cleaner, more efficient, and easier to maintain. By following these best practices, you can streamline your development process, reduce duplication, and build applications that are robust, scalable, and easy to extend.