How to Build a Restful Api with Lumen
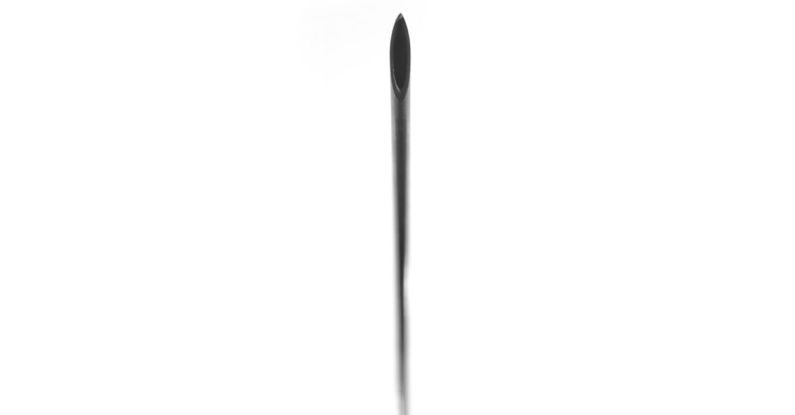
Building a RESTful API with Lumen
Lumen, a micro-framework by Laravel, provides a seamless way to create RESTful APIs. With its lightweight and fast performance, Lumen is an excellent choice for developing APIs. In this article, we will explore how to build a RESTful API with Lumen in a few simple steps.
Setting Up Your Lumen Project
To start building a RESTful API with Lumen, the first step is to set up your Lumen project. Make sure you have Composer installed on your system. You can create a new Lumen project using the following Composer command:
“`
composer create-project –prefer-dist laravel/lumen project-name
“`
Once the project is created, navigate to the project directory and run the built-in PHP development server using the following command:
“`
php -S localhost:8000 -t public
“`
Defining Routes
Routes in Lumen are defined in the `routes/web.php` file. You can define routes for various HTTP methods such as GET, POST, PUT, PATCH, and DELETE. Here is an example of defining a simple GET route:
“`php
$router->get(‘/api/users’, function () use ($router) {
return ‘List of users’;
});
“`
This route will return the message ‘List of users’ when accessed via a GET request to `/api/users`.
Creating Controllers
Controllers in Lumen handle the application’s logic and interact with the database. You can create a new controller using the Artisan command:
“`
php artisan make:controller UserController
“`
This command will create a new UserController class in the `app/Http/Controllers` directory. You can define methods in the controller to handle different API endpoints.
Creating Models and Migrations
Models in Lumen represent the data structure of your application. You can create a new model using the following Artisan command:
“`
php artisan make:model User
“`
This command will create a new User model in the `app` directory. You can also generate a migration file for the User model using the command:
“`
php artisan make:migration create_users_table
“`
This command will create a new migration file in the `database/migrations` directory. You can define the schema of the users table in this migration file.
Connecting to the Database
Lumen uses the Eloquent ORM to interact with the database. You need to configure the database connection in the `.env` file located in the project root directory. Update the database configuration variables with your database credentials.
“`env
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=root
DB_PASSWORD=secret
“`
Once the database configuration is set up, you can run the database migrations to create the users table in the database:
“`
php artisan migrate
“`
Implementing CRUD Functionality
To implement CRUD functionality in your API, you can define routes, controllers, and models for creating, reading, updating, and deleting resources. Here is an example of defining routes for a CRUD API:
“`php
$router->get(‘/api/users’, ‘UserController@index’);
$router->post(‘/api/users’, ‘UserController@store’);
$router->put(‘/api/users/{id}’, ‘UserController@update’);
$router->delete(‘/api/users/{id}’, ‘UserController@delete’);
“`
In the UserController, you can define methods like `index`, `store`, `update`, and `delete` to handle the corresponding API endpoints.
Securing Your API
To secure your API, you can implement authentication and authorization mechanisms in Lumen. You can use middleware to restrict access to certain routes based on user roles or permissions. Lumen provides a simple way to define middleware and apply them to routes.
Testing Your API
Once you have implemented your RESTful API with Lumen, it is essential to test it thoroughly. You can use tools like Postman to send HTTP requests to your API endpoints and verify the responses. Make sure to test different scenarios, including success and error cases.
In conclusion,
Building a RESTful API with Lumen is a straightforward process that involves setting up the project, defining routes, creating controllers and models, connecting to the database, implementing CRUD functionality, securing the API, and testing it thoroughly. By following these steps, you can create a robust and efficient API using Lumen.